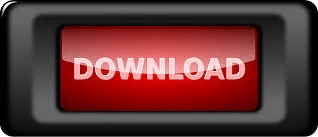

- VB NET 2010 CONSOLE APPLICATION CHECK INPUT IS LIKE STRING HOW TO
- VB NET 2010 CONSOLE APPLICATION CHECK INPUT IS LIKE STRING CODE
If you think about it carefully, the algorithm you have used to convert from denary to binary can be generalised.
VB NET 2010 CONSOLE APPLICATION CHECK INPUT IS LIKE STRING HOW TO
The main reason to still write the additional functions is so that you know how to do it. You could convert it to denary using the functions that you have made and then call the denary to binary function to do the business for you again. You may have spotted that a quicker solution is to call the functions that you already have. You write another function which calls this for each of the characters in the input string, adding them onto a string until the whole number has been represented. You write a function that accepts a hex character and returns the equivalent 4 bit binary pattern. The easiest way to program the conversion from hex to binary is to do it is to convert a character at a time. For each of the sixteen permissibles digits in hex (0-15), there is a single 4 bit binary pattern. The two missing features are clearly the conversions between hex and binary. The program so far should convert successfully between denary and either hex or binary.
/GettyImages-174616627-5769ec8e5f9b58346a84bfbf.jpg)
Adding Conversions Between Hex And Binary You may find it useful to write a function to convert a single digit from hex to decimal and another to convert the other way. You will need to convert the digit into an integer by checking whether what it currently contains. You will need to convert the string to an integer. When converting from Hex, instead of calculating the columns place value by finding the power of 2, use a power of 16. You will need to examine the result in order to store the correct character (0 - 9 + A, B, C, D, E, F) in the myAnswer String (use if or select case statements). When converting to Hex, instead of dividing by 2, divide by 16. The functions should mirror the ones above. Return myAnswer Conversion To And From Hex MyAnswer ← myAnswer + (thisBit * thisPlaceValue) ThisPlaceValue ← Int(Math.Pow (2.0f, CDbl(i)) Conversion From Denary To Binary Declare string array bits = new stringĭeclare string myAnswer ← String.Join("", bits)Ĭonversion From Binary To Denary Declare int myAnswer ← 0ĭeclare string reversed ← StrReverse(inputString) Dry-running helps to show you how values stored in the variables change in the program and come to equal the desired result. Writing a program flowchart can help you visualise things better. The key is to also try to understand how the algorithm achieves the result that it does. When you implement an algorithm from someone else's pseudocode, the easy part of the exercise is translating the programming structures that you see into the syntax demanded by the Visual Basic.NET compiler.
VB NET 2010 CONSOLE APPLICATION CHECK INPUT IS LIKE STRING CODE
Th following pseudocode and explanations should help you to write fill in the missing code in the program. 'procedure to wait for user input before stoppingĬonsole.WriteLine("Press any key to continue")įunction DenToBin(ByVal inputString As String) As Stringįunction BinToDen(ByVal inputString As String) As Integerįunction DenToHex(ByVal inputString As String) As Stringįunction HexToDen(ByVal inputString As String) As Integer 'function to display menu and read in user choiceĬonsole.WriteLine("Binary And Hex Conversion Program")Ĭonsole.WriteLine("*********************************")ĭim menChoice As Integer = Console.ReadLine()Ĭonsole.Write("Enter the value to convert: ")
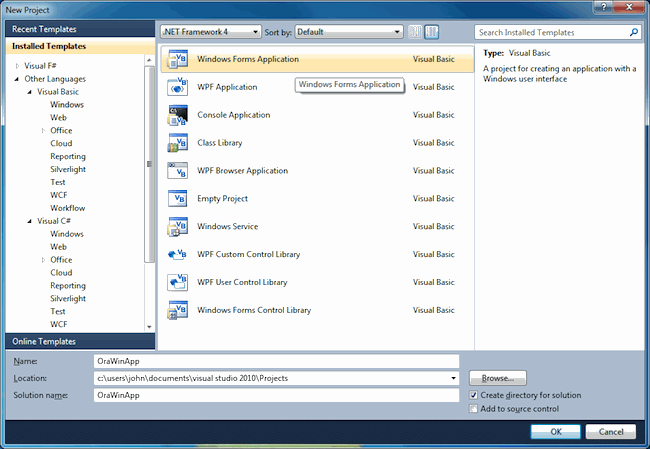
It is relatively easy to set your programs up like this if you used a hierarchy chart to plan what you were going to do.ĭim menuchoice As Integer = GetMenuChoice()Ĭonsole.WriteLine("", strCode, HexToDen(strCode)) The key functions have not been programmed yet. The following code shows the structure of the program. You need to create a new console application in Visual Basic. This page provides you with instructions on how to set up a program to perform conversion between different number bases.
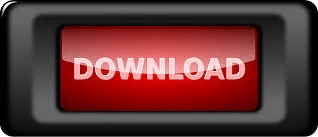